Ready to spot the International Space Station from your backyard? This tutorial will show you how to predict the next ISS flyover using Python. Before we dive in, tune into this Google-powered podcast episode to get the lowdown on what makes this project so exciting:
Determine the Coordinates for Your Location
To calculate when the ISS flies over your head (or is at least theoretically visible), you first need the coordinates for your location. The website latlong.net is suitable for this – enter your location here and you’ll immediately get the corresponding coordinates:
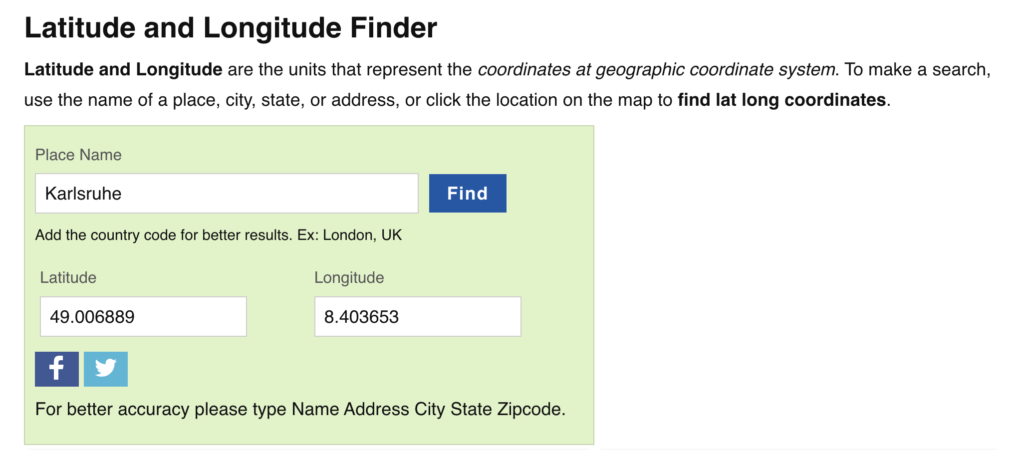
In the above case, that would be approximately 49° North latitude and 8.4° East longitude for Karlsruhe in the south of Germany. You’ll need these numbers later in the Python script to calculate the flyover or visibility of the ISS.
The Required Python Library
To determine the ISS flyover, you need the ephem library, which you install in the terminal as follows:
pip install ephem
___STEADY_PAYWALL___
This library offers extensive functions for astronomical observations – you can use it to calculate the trajectories of various celestial bodies such as planets, asteroids, and comets, as well as satellites, which in the broadest sense includes the ISS. You can learn more about this great project on the official website.
Obtaining Current TLE Data
For the ephem library to calculate the ISS flyover, it needs current data of the space station in addition to your location. This is where a so-called Two-Line Element, or TLE for short, comes into play. This is a standardized format for a series of data of a flying object in Earth’s orbit. You can learn more about TLEs on Wikipedia.
To obtain the current TLE of the ISS, the two websites from ARISS and Celestrak are suitable. On both sites, you’ll find the current data for the ISS module Zarya. Here’s an example of a TLE:
ISS (ZARYA)
1 25544U 98067A 24238.20528372 .00032948 00000-0 58135-3 0 9999
2 25544 51.6406 333.3912 0006392 271.4643 173.4301 15.50058100469255
You can copy these two lines from the website and enter them directly into your script. An important note: The TLE for the space station is regularly updated and adjusted to the actual conditions of the ISS. To ensure your calculation provides reliable data, it’s best to copy the current values into your script.
Calculate the Next ISS Flyover
Now to the core of the project: you can calculate the next ISS flyover with the following Python script:
import ephem
# Your coordinates
latitude = '49'
longitude = '8.4'
# Current TLE
tle1 = "1 25544U 98067A 24238.20528372 .00032948 00000-0 58135-3 0 9999"
tle2 = "2 25544 51.6406 333.3912 0006392 271.4643 173.4301 15.50058100469255"
# Instance for your location
observer = ephem.Observer()
observer.lat = latitude
observer.lon = longitude
# Instance for the ISS
iss = ephem.readtle("ISS (ZARYA)", tle1, tle2)
# Height of your location (optional) and local time
observer.elevation = 0 # Elevation in meters (optional)
observer.date = ephem.localtime(ephem.now()) # Use current date and time
print("Current time (local):", observer.date)
# Calculate next flyover
next_pass = observer.next_pass(iss)
# Print the result
print("Next pass of the ISS:")
print(f"Date and Time (local time): {ephem.localtime(next_pass[0])}")
print(f"Rise (local time): {ephem.localtime(next_pass[1])}")
print(f"Maximum Elevation (local time): {ephem.localtime(next_pass[2])}")
print(f"Set (local time): {ephem.localtime(next_pass[3])}")
print(f"Max Elevation (degrees): {next_pass[4]}")
How the Script Works
After importing the ephem library, you enter the coordinates of your location. Then follow the values of the current TLE. Next, you create an observer instance that contains the coordinates of your location, as well as an instance for the ISS with the TLE values.
In addition to the coordinates, you can also enter the elevation of your location. If it’s 150 meters above sea level, for example, simply enter 150 here. In the line below, you set the current time of your time zone.
With all this data, you call the function observer.next_pass(iss) and store the result in the variable next_pass. The calculation thus takes into account your location data in the observer instance as well as the TLE values in the iss instance.
Finally, you just need to output the results. However, there’s a small problem here: Actually, you don’t just get one point in time when the ISS is visible in the sky, but also the time when it rises above the horizon and disappears behind it again. Additionally, ephem also calculates the maximum angle of the ISS above the horizon. Unfortunately, at least in my tests, there’s only one meaningful value: the time of its maximum height:
Next pass of the ISS:
Date and Time (local time): 2024-08-26 02:50:05.657860
Rise (local time): 1900-01-03 17:40:39.115906
Maximum Elevation (local time): 2024-08-26 02:54:23.887056
Set (local time): 1899-12-31 16:40:30.704498
Max Elevation (degrees): 2024/8/26 00:58:43
As you can see in the above output, the time indications for Rise and Set are nonsensical. The same goes for the Max Elevation value. However, you can find a good (and possibly sufficient for your project) value behind Maximum Elevation (local time) – this time indication tells you when the next ISS flyover will be visible at your location.
If you have a clue or a solution for these errors, feel free to write to me at info@polluxlabs.net – I’m grateful for any hints.
Verification of Results
If you’re still not quite convinced by the calculation, you can, for example, use an app on your smartphone to check where the space station is currently located and when you can expect the next ISS flyover. I personally use the free app SkyView Lite for this.
What Can You Build with This?
If you “only” need the time for the next ISS flyover so you can observe it in the evening or morning sky, this script is probably enough for you. However, if you want to build something, a LEGO ISS might be interesting for you – put a small Raspberry Pi in it and let it glow when the ISS is above you!
Here’s what the illuminated model could look like:
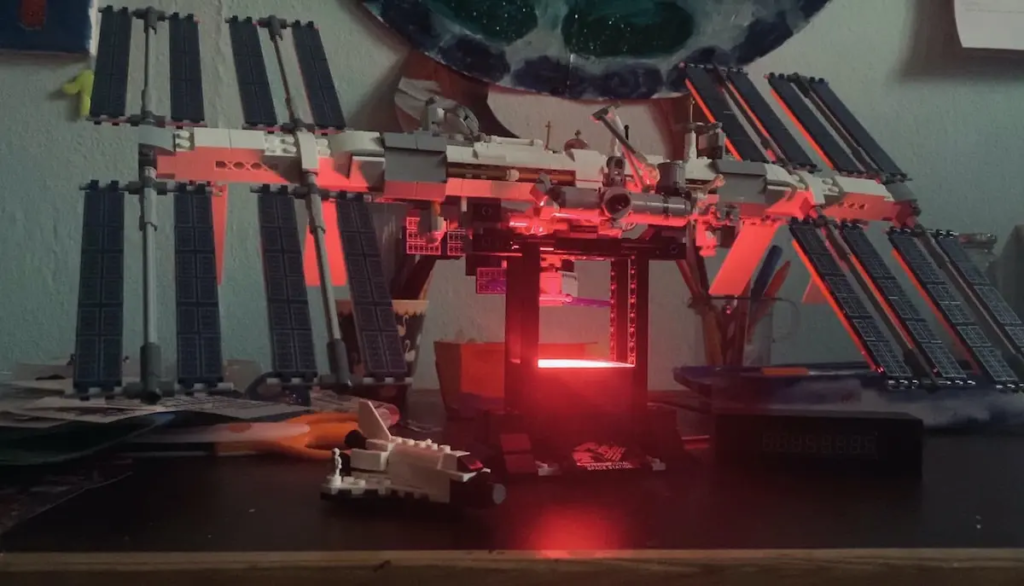