In this project, you’ll build your own Arduino alarm system. It consists of three components: a sound sensor, an active piezo buzzer, and an RGB LED.
You’ll use the sound sensor to measure the ambient noise level. When certain thresholds set by you are exceeded or not met, the RGB LED will light up green or yellow. If the noise level exceeds a specified limit, the LED will turn red, and the piezo will emit a shrill alarm tone.
In the following sections, you’ll learn how to connect the sound sensor, the piezo buzzer, and the RGB LED. Then you’ll see how all three components work together to form the Arduino alarm system.
The Sound Sensor
First, you’ll learn how to connect the sound sensor (KY-037) and process sounds both analog and digital in your Arduino UNO.
The main components of the sound sensor you’ll be working with are: the microphone, a comparator (here the LM393, which compares two voltages), and a potentiometer (to set the threshold). You’ll connect the sensor using at least 3 of the 4 pins – anode, cathode, analog and/or digital output.
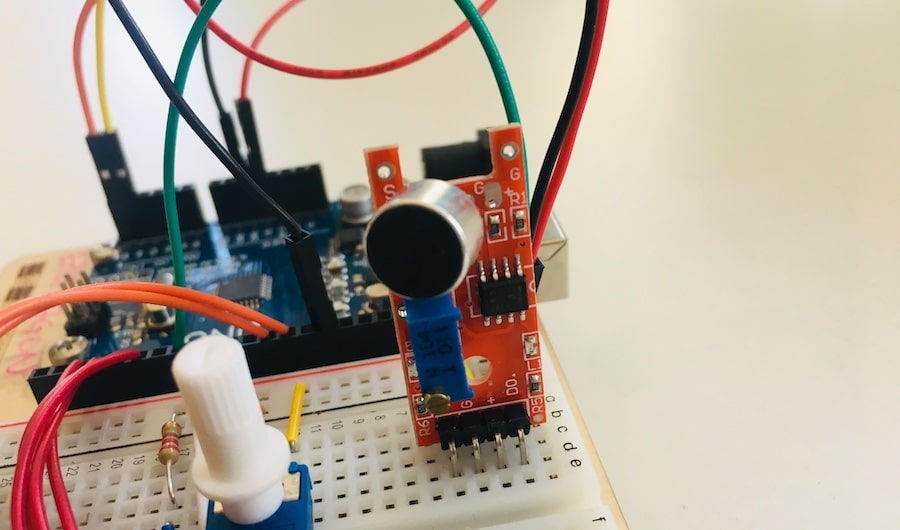
Connecting the Digital Output
You can connect the sound sensor to your Arduino in two ways – analog or digital. The digital connection is useful when you want to trigger something as soon as a loud noise is detected. This could be, for example, a knock on the door or a bang. Here’s how to connect your sensor:
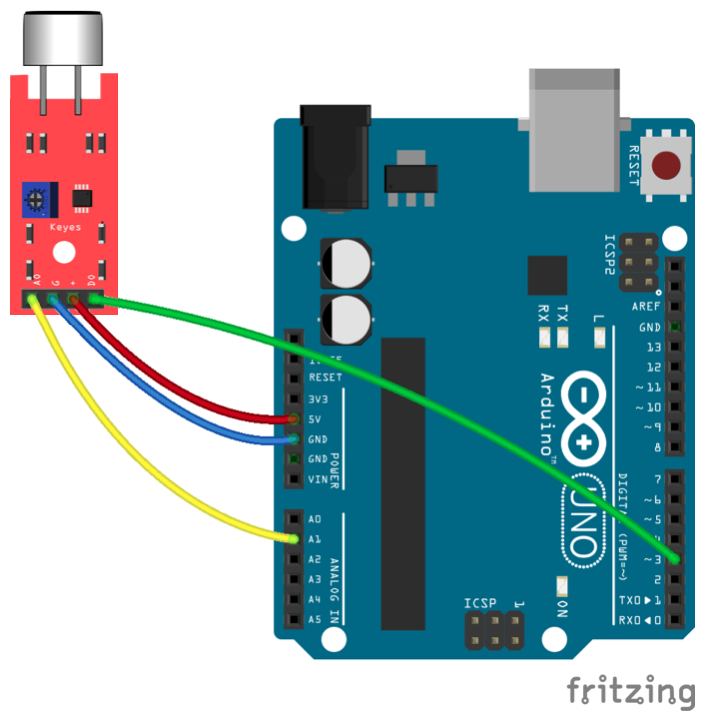
Power your sensor via the + and G (for Ground, i.e., minus) pins and connect the digital output (DO) to the Arduino’s digital input 3. Connect the analog output (AO) to the Arduino’s pin A1. And that’s it.
Now copy the following sketch and upload it to your Arduino UNO.
const int sensor = 3;
const int led = LED_BUILTIN;
int noise = 0;
void setup() {
pinMode(sensor, INPUT);
pinMode(led, OUTPUT);
Serial.begin(9600);
}
void loop() {
noise = digitalRead(sensor);
Serial.println(noise);
if (noise == 1){
digitalWrite(led, HIGH);
}else{
digitalWrite(led, LOW);
}
}
If the Arduino’s internal LED is now constantly lit, it means the sensor is continuously detecting a sound. Now take a small screwdriver and turn the screw on the potentiometer to the left – until the LED goes out.
Make a loud noise right next to the microphone – for example, snap your fingers. The LED should light up briefly. If not – turn the screw slightly to the right again. With a little sensitivity, you’ll find the right fine adjustment. You can also follow in the Serial Monitor whether the sensor detects a sound: In “silence” you’ll see a zero there, with a sound a 1.
And Now the Analog Output
When you connect the sound sensor analog, you get real-time feedback on the volume. For example, you can make an LED light up as soon as a volume you specify is exceeded.
You’ve already connected the sensor’s analog output to your Arduino. Now upload the following sketch.
const int sensor = A1;
const int led = LED_BUILTIN;
int noise = 0;
void setup() {
pinMode(sensor, INPUT);
pinMode(led, OUTPUT);
Serial.begin(9600);
}
void loop() {
noise = analogRead(sensor);
Serial.println(noise);
if (noise > 200){
digitalWrite(led, HIGH);
}else{
digitalWrite(led, LOW);
}
}
To better track the volume, start the Serial Monitor and observe the sensor values there. Some fine-tuning is needed again here. Adjust the potentiometer so that you see values in the serial monitor that are just below 200. Now if you make a noise that’s loud enough to make the value jump above 200, the Arduino’s internal LED will light up.
So you see, it’s not enough here for a sound to be detected. This sound must also be loud enough to turn on the LED.
You’ll represent the volume with an RGB LED, and once a certain volume is exceeded, an alarm signal will sound from the piezo buzzer.
Connecting the Active Piezo and the RGB LED
You’ve already installed the sound sensor. However, for the Arduino alarm system, you only need the analog output (AO) of the sensor. You can omit or remove the cable on the digital output.
You’ve already gotten to know the passive piezo buzzer with the theremin. As you know, you can generate different tones with this. Not so with the active piezo: This component can only generate a single tone – and does so as soon as it’s supplied with power. However, this is perfectly sufficient for an alarm system.
If you’re not sure whether your piezo is active or passive, simply apply 5V voltage directly from your Arduino. Does a tone sound? Then it’s the active piezo you need for this project.
The connection is very simple. Connect the short leg of the piezo buzzer to minus and the long one to digital pin 4 on your Arduino. Later, when the sound sensor triggers an alarm, you’ll route power to the piezo through this pin, which will then start to beep.
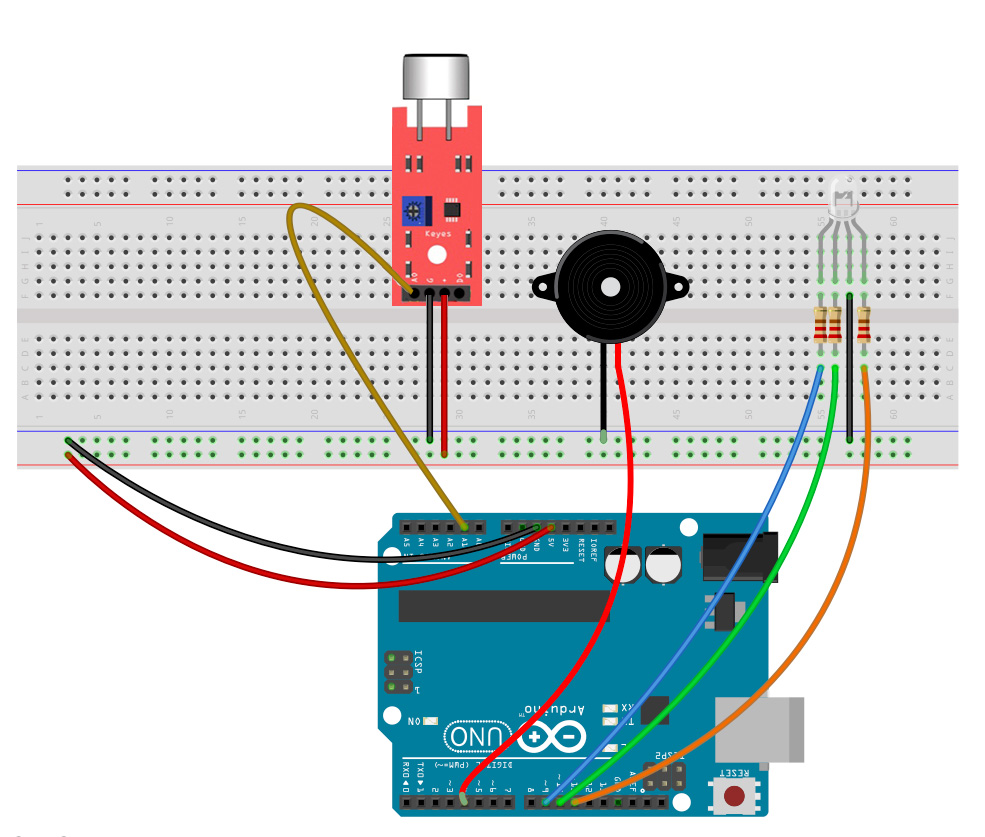
Connecting the RGB LED is a bit more complex. It has a total of 4 legs: a cathode, which you connect to minus, and three anodes – one each for the colors red, green, and blue. As you’re used to with “regular” LEDs, you also need to install a 220Ω current-limiting resistor here – one for each anode.
Connect the three anodes to digital pins 9, 10, and 11. These three pins have pulse width modulation (PWM), which you’ve already learned about when you controlled the brightness of a simple LED.
The color channels and Arduino pins shown above may not match depending on the design of your LED. In the example below, the red color channel is to the right of the cathode, which may be different for your LED. However, you can easily figure this out and fix it later when you switch the color of the light: Simply adjust the variables in the sketch and write the correct pin behind the color channel:
int ledRed = 10;
int ledGreen = 9;
int ledBlue = 8;
If you’ve built and wired everything as shown in the sketch above, you can move on to the code.
The Sketch for the Arduino Alarm System
Now that you’ve built everything on your breadboard, it’s time for the program. In the following, you’ll learn more about the individual parts of the program and their function.
Right at the beginning of the sketch, you define which hardware you’ve connected to which pins. Of course, you can also define these as constants with const. You also need a few variables for the brightness of the individual color channels of the RGB LED (e.g., brightnessRed = 150) and the volume measured by the sound sensor. You initially set these to zero.
int ledRed = 11;
int ledGreen = 10;
int ledBlue = 9;
int brightnessRed = 150;
int brightnessGreen = 150;
int brightnessBlue = 150;
int noise = 0;
int sensor = A1;
int piezo = 4;
The Setup Function
There’s probably nothing here that you don’t already know. You set the pins of the LED and the piezo as OUTPUT and start the Serial Monitor.
void setup() {
pinMode(ledRed, OUTPUT);
pinMode(ledGreen, OUTPUT);
pinMode(ledRed, OUTPUT);
pinMode(piezo, OUTPUT);
Serial.begin(9600);
}
The Loop Function
This is where it gets exciting. First, you measure the ambient noise level, because all other actions in the sketch later are based on this – i.e., whether your Arduino alarm system triggers or not.
noise = analogRead(sensor);
Serial.println(noise);
Then comes the first conditional statement. If the noise level is below a certain value, the LED should light up green – which means as much as “All clear”. You can of course adjust the value of 200 (and the following ones in the further instructions). Also make sure that when you first start the Arduino alarm system, you calibrate the sound sensor so that it’s below the value you’ve set when it’s quiet.
if(noise <= 200){
analogWrite(ledGreen, brightnessGreen);
analogWrite(ledRed, 0);
analogWrite(ledBlue, 0);
digitalWrite(piezo, LOW);
}
As mentioned, in this state, the LED should light up green. You achieve this by only lighting up the green color channel with the brightness you’ve set, brightnessGreen. The other two channels for Red and Blue receive the brightness zero, so they’re off. Of course, you can also mix colors (even if the LED can’t display them very clear) – for this purpose, this RGB Color Wheel is suitable, for example.
The piezo shouldn’t sound here either, which is why you don’t supply power to it. You achieve this with the LOW parameter in the digitalWrite() function.
Another Instruction
Now if the noise level rises somewhat, but is still not high enough for an alarm, a second conditional statement comes into play – with else if{}.
else if(noise > 200 && noise <= 350){
analogWrite(ledRed, brightnessRed);
analogWrite(ledGreen, brightnessGreen);
analogWrite(ledBlue, 0);
digitalWrite(piezo, LOW);
}
These commands are executed when the volume is between 201 and 350. As mentioned, experiment with these values to adjust them to your circumstances.
So if the volume is in this range, the LED light changes from green to yellow. There’s no separate color channel for this color, which is why you simply mix it. Yellow is a mixture of red and green. Therefore, you turn on these color channels of the LED and let the blue channel go out.
Alarm!
If it now gets even louder, the alarm should sound. The LED shines in a rich red and the piezo buzzer starts to beep.
else if(noise > 350){
analogWrite(ledRed, brightnessRed);
analogWrite(ledGreen, 0);
analogWrite(ledBlue, 0);
digitalWrite(piezo, HIGH);
delay(10000);
}
This time, you only turn on the red channel of the LED. You also now route power from the Arduino to the piezo – with the function digitalWrite(piezo, HIGH);
Certainly, an alarm system should make noise until it’s turned off. However, for a first experiment, maybe one second is enough for now. That’s why there’s a delay() of 1,000 milliseconds at the end.
And that’s it! Upload the following sketch to your Arduino, calibrate your sound sensor, and try out your Arduino alarm system.
//Arduino Alarm System
//en.polluxlabs.net
int ledRed = 11;
int ledGreen = 10;
int ledBlue = 9;
int brightnessRed = 150;
int brightnessGreen = 150;
int brightnessBlue = 150;
int noise = 0;
int sensor = A1;
int piezo = 4;
void setup() {
pinMode(ledRed, OUTPUT);
pinMode(ledGreen, OUTPUT);
pinMode(ledRed, OUTPUT);
pinMode(piezo, OUTPUT);
Serial.begin(9600);
}
void loop() {
noise = analogRead(sensor);
Serial.println(noise);
if(noise <= 200){
analogWrite(ledGreen, brightnessGreen);
analogWrite(ledRed, 0);
analogWrite(ledBlue, 0);
digitalWrite(piezo, LOW);
}
else if(noise > 200 && noise <= 350){
analogWrite(ledRed, brightnessRed);
analogWrite(ledGreen, brightnessGreen);
analogWrite(ledBlue, 0);
digitalWrite(piezo, LOW);
}
else if(noise > 350){
analogWrite(ledRed, brightnessRed);
analogWrite(ledGreen, 0);
analogWrite(ledBlue, 0);
digitalWrite(piezo, HIGH);
delay(10000);
}
}