In this project, you’ll build a Arduino Code Lock using a membrane keypad and a servo motor. Only by entering the correct combination will the servo motor move and potentially release a lock.
Connecting the Membrane Keypad
For a code lock, you naturally need a device to enter the code. The 4×4 membrane keypad is perfect for this purpose.
First, install the connection to the Arduino. The keypad has 8 sockets; insert the same number of cables and connect them in order to the digital pins 9 to 2 on your Arduino:
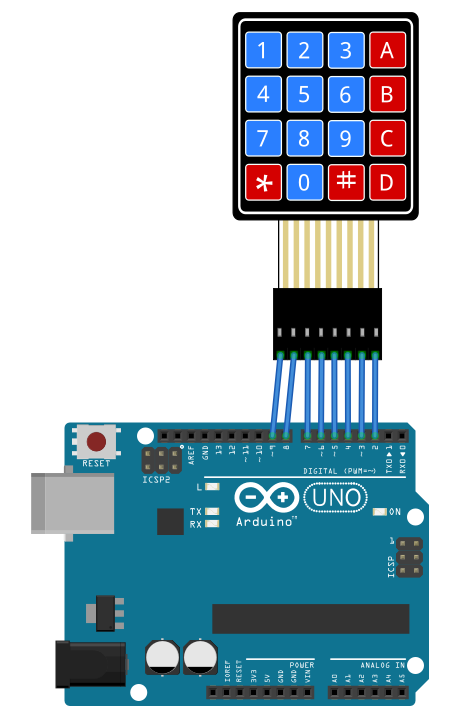
The Keypad Library
To make controlling the keypad as easy as possible, there’s a library available. This time, however, you won’t use the Library Manager, but include the library manually. First, download it here.
Now select Sketch -> Include Library -> Add .ZIP Library in the Arduino IDE menu.
For an initial test of the keypad, use the example sketch that comes with the library. Open it under File -> Examples -> Keypad -> CustomKeypad
Before we look at the code, first upload the sketch to your Arduino. Now open the Serial Monitor and press one of the keys on the keypad. Does the corresponding character appear in the output? If so, perfect.
The Example Sketch
Let’s take a very quick look at this sketch. Most of the code consists of functions from the Keypad library, but two things are particularly interesting. First, you see in the code the number of keys per row and column, as well as a matrix that determines the values or characters of the keypad:
const byte ROWS = 4;
const byte COLS = 4;
char hexaKeys[ROWS][COLS] = {
{'1','2','3','A'},
{'4','5','6','B'},
{'7','8','9','C'},
{'*','0','#','D'}
};
These are the characters you also find on the keypad itself. If you ever want to process different characters, you could enter them here and thus assign them to a key on the membrane keypad.
The connection of the keypad to the Arduino is hidden in these two variables:
byte rowPins[ROWS] = {9, 8, 7, 6};
byte colPins[COLS] = {5, 4, 3, 2};
___STEADY_PAYWALL___
These are the pins you also find in the connection diagram above. If you ever need to use one of these pins for another component, you can assign a different digital pin to the keypad here.
Also, the customKeypad object is created with these parameters:
Keypad customKeypad = Keypad( makeKeymap(hexaKeys), rowPins, colPins, ROWS, COLS);
Let’s move on – next, you’ll install two LEDs and the servo motor and make sure it only moves after entering the correct code.
Setting Up and Programming the Arduino Code Lock
First, connect your servo motor to the Arduino. You can either install it on your breadboard and connect from there – or you can plug the cables of the servo directly into the pins GND, 5V, and 11, as shown in this sketch:
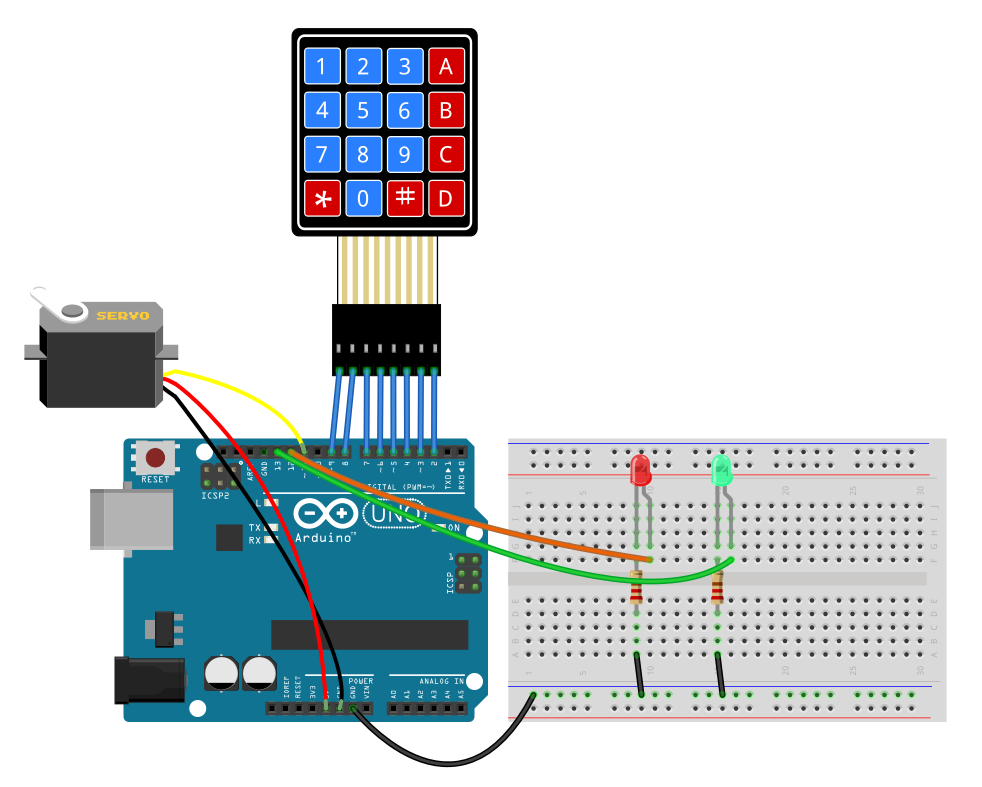
The order of the connections GND, VCC, and Signal may vary depending on the make of the motor. Please pay attention to this when connecting.
In addition, there are two LEDs with two 220Ω resistors each. Connect these to digital pins 12 and 13. These will later indicate whether the code was entered correctly or incorrectly.
The Sketch for Your Arduino Code Lock
This brings us to the core of the project. The servo should only move when someone enters the correct code via the keypad. Then the pointer turns to the side and releases, for example, the lid of a box. How you design the housing of the box is, of course, up to you.
At the beginning of the sketch, as usual, first include the required libraries:
#include <Keypad.h>
#include <Servo.h>
Then follow the information for the keypad that you already know:
const byte ROWS = 4;
const byte COLS = 4;
char hexaKeys[ROWS][COLS] = {
{'1', '2', '3', 'A'},
{'4', '5', '6', 'B'},
{'7', '8', '9', 'C'},
{'*', '0', '#', 'D'}
};
byte rowPins[ROWS] = {9, 8, 7, 6};
byte colPins[COLS] = {5, 4, 3, 2};
Keypad customKeypad = Keypad( makeKeymap(hexaKeys), rowPins, colPins, ROWS, COLS);
Now you need three variables or constants that contain the pressed key, the entire entered code inputCode, and the password code you’ve set. You’ll check the input against the latter later. If you want to set your own code later, you can do it here.
char key;
String inputCode;
const String code = "1103A"; // The code you set
Finally, you need an object for the servo motor and the two variables for the LED connections.
Servo servo;
int redLED = 12;
int greenLED = 13;
The Setup Function
Here you start the Serial Monitor, set the pinMode for the LEDs, and the connection pin of the servo motor.
void setup() {
Serial.begin(9600);
pinMode(redLED, OUTPUT);
pinMode(greenLED, OUTPUT);
servo.attach(11);
}
The Loop
First, you need a variable to store the value of the key that was just pressed. This is the variable key of type char for character. You store the value using the function customKeypad.getKey().
char key = customKeypad.getKey();
Now follows a series of conditional statements. The Arduino Code Lock uses two keys to lock the lock (with the * key) or to check the input (with #). So you must not use these keys in your stored code. The first if statement “listens” for the * key and locks the lock by setting the servo to an angle of 90°. Of course, you can change this angle according to your needs.
Additionally, it turns on the red LED (and turns off the green one) and clears the inputCode variable so that no “old” characters are left here for the next input.
if (key == '*') {
inputCode = "";
Serial.println("Lock engaged.");
delay(1000);
servo.write(90);
digitalWrite(greenLED, LOW);
digitalWrite(redLED, HIGH);
The second query follows immediately with an else if and is triggered when the # key is pressed. In this case, two more queries follow – one that is executed when the code was entered correctly and the other if it was not.
} else if (key == '#') {
if (inputCode == code) {
Serial.println("The code is correct. Opening the lock...");
digitalWrite(greenLED, HIGH);
digitalWrite(redLED, LOW);
servo.write(0);
} else {
Serial.println("The code is incorrect!");
digitalWrite(greenLED, LOW);
digitalWrite(redLED, HIGH);
}
If the code is correct, i.e., inputCode == code is true, the green LED lights up and the servo moves to 0°. If the input is incorrect, the red LED lights up accordingly – and nothing moves. In both cases, the variable for the entered code is cleared again:
inputCode = "";
Now you have everything to lock the Arduino Code Lock at the push of a button. Also to check whether the code is right or wrong and react accordingly. But how is the inputCode variable actually filled?
This happens in a final else – the instruction that is executed for all keys except * and #. Here you simply add the last pressed character to the inputCode variable:
} else {
inputCode += key;
Serial.println(inputCode);
}
Here’s the complete sketch – enjoy your new Arduino Code Lock! 🙂
//Arduino Code Lock Project
//en.polluxlabs.net
#include <Keypad.h>
#include <Servo.h>
const byte ROWS = 4;
const byte COLS = 4;
char hexaKeys[ROWS][COLS] = {
{'1', '2', '3', 'A'},
{'4', '5', '6', 'B'},
{'7', '8', '9', 'C'},
{'*', '0', '#', 'D'}
};
byte rowPins[ROWS] = {9, 8, 7, 6};
byte colPins[COLS] = {5, 4, 3, 2};
Keypad customKeypad = Keypad( makeKeymap(hexaKeys), rowPins, colPins, ROWS, COLS);
char key;
String inputCode;
const String code = "1103A"; // The code you set
Servo servo;
int redLED = 12;
int greenLED = 13;
void setup() {
Serial.begin(9600);
pinMode(redLED, OUTPUT);
pinMode(greenLED, OUTPUT);
servo.attach(11);
}
void loop() {
char key = customKeypad.getKey();
if (key) {
if (key == '*') {
inputCode = "";
Serial.println("Lock engaged.");
delay(1000);
servo.write(90);
digitalWrite(greenLED, LOW);
digitalWrite(redLED, HIGH);
} else if (key == '#') {
if (inputCode == code) {
Serial.println("The code is correct. Opening the lock...");
digitalWrite(greenLED, HIGH);
digitalWrite(redLED, LOW);
servo.write(0);
} else {
Serial.println("The code is incorrect!");
digitalWrite(greenLED, LOW);
digitalWrite(redLED, HIGH);
}
inputCode = "";
} else {
inputCode += key;
Serial.println(inputCode);
}
}
}