Have you ever wanted to know the temperature and humidity levels in your home or garden with precision? With this DIY Arduino weather station project, you can create your own customized environmental monitoring system. This beginner-friendly project combines the versatility of an Arduino board with affordable sensors to give you accurate, real-time climate data.
In this tutorial, you’ll learn how to:
- Set up an Arduino Uno with a DHT11 temperature and humidity sensor
- Connect an LCD to show readings
- Write Arduino code to take measurements and update the display
- Expand the project with additional sensors and features
Let’s dive in and start building your very own Arduino weather station!
Components You’ll Need:
- Arduino Uno board
- DHT11 temperature and humidity sensor
- 16×2 LCD
- 10k ohm potentiometer
- Breadboard
- Jumper wires
Setting Up the Hardware
Your breadboard will soon be filled with numerous wires. Use the following diagram as a guide when assembling your Arduino weather station:
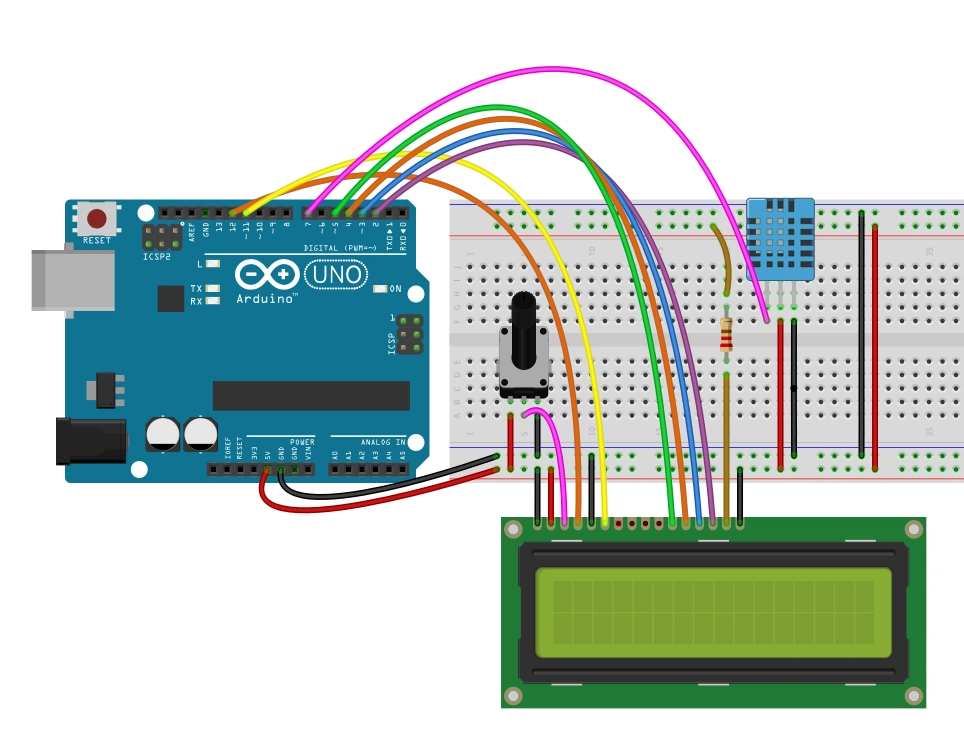
Your hardware setup is now complete! Let’s move on to the next preparation:
Installing the DHT11 Library
To use the DHT11 sensor with your Arduino, you’ll need to install a specific library. The DHT library provides an easy-to-use interface for reading temperature and humidity data from DHT sensors, including the DHT11.
Here’s how to install the DHT library:
- Open the Arduino IDE
- Go to Sketch > Include Library > Manage Libraries
- In the Library Manager, search for “DHT sensor library”
- Look for the library by Adafruit and click “Install”
- If prompted, also install any dependencies (like the Adafruit Unified Sensor library)
Why the DHT Library is Necessary
The DHT library is essential for this project for several reasons:
- Simplified Communication: The DHT11 uses a specific single-wire protocol to communicate. The library handles all the low-level timing and communication details, allowing you to focus on using the data rather than figuring out how to retrieve it.
- Error Handling: The library includes built-in error checking to ensure that the data received from the sensor is valid.
By using this library, you’ll save time and avoid common pitfalls associated with directly interfacing with the sensor. This allows you to get your Arduino weather station up and running quickly and reliably.
___STEADY_PAYWALL___
The Arduino Sketch
Here’s the code for your Arduino weather station. Copy this into your Arduino IDE:
//Arduino Weather Station
//en.polluxlabs.net
#include <LiquidCrystal.h>
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
#include "DHT.h"
#define DHTPIN 7
#define DHTTYPE DHT11 //or DHT22 if you use it
DHT dht(DHTPIN, DHTTYPE);
float temp;
float humidity;
void setup() {
Serial.begin(9600);
lcd.begin(16, 2);
dht.begin();
}
void loop() {
temp = dht.readTemperature();
humidity = dht.readHumidity();
Serial.print("Temperature: ");
Serial.print(temp);
Serial.println(" *C");
Serial.print("Humidity: ");
Serial.print(humidity);
Serial.println(" %");
Serial.println();
//Print Temperature
lcd.setCursor(0, 0);
lcd.print(temp + String(" C"));
//Primt Humidity
lcd.setCursor(0, 1);
lcd.print(humidity + String(" %"));
delay(10000);
}
Understanding the Code
Let’s break down the key parts of this sketch:
- We include the necessary libraries for the LCD and DHT sensor.
- We define the pin the DHT11 is connected to and specify its type.
- We create objects for the DHT sensor and LCD display.
- In the
setup()
function, we initialize the LCD and DHT sensor. - In the
loop()
function:- We wait for 2 seconds between readings to not overload the sensor.
- We read the humidity and temperature from the DHT11.
- We check if the readings are valid.
- We clear the LCD and display the new temperature and humidity values.
Uploading and Testing
- Connect your Arduino to your computer via USB.
- Open the Arduino IDE and paste in the code above.
- Select your Arduino board and port from the Tools menu.
- Click the Upload button to send the code to your Arduino.
If everything is set up correctly, you should now see the temperature and humidity readings on your LCD, updating every 2 seconds!
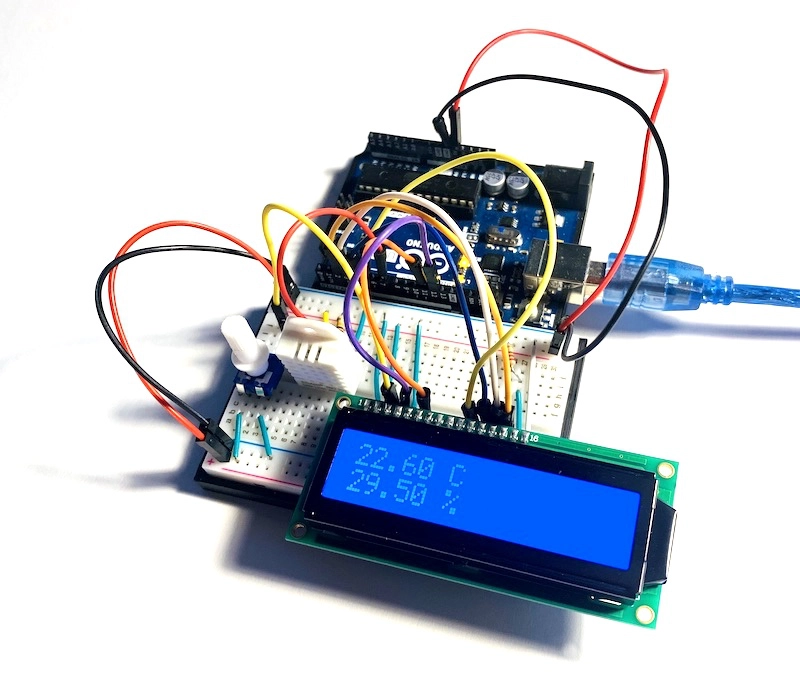
Troubleshooting
- If you see “DHT11 error!” on the display, check your wiring connections to the sensor.
- If the LCD is blank, adjust the potentiometer to change the contrast.
- Ensure you’ve installed the DHT library in your Arduino IDE (Sketch > Include Library > Manage Libraries).
Expanding Your Weather Station
Now that you have a basic weather station working, here are some ideas to expand its capabilities:
- Add a pressure sensor like the BMP280 to measure atmospheric pressure.
- Incorporate a light sensor to measure ambient light levels.
- Connect a data logging shield to record measurements over time.
- Add a Wi-Fi module to send data to a web server or IoT platform.
- Include a rain gauge or anemometer for more comprehensive weather data.
Conclusion
Congratulations! You’ve built your own Arduino weather station. This project not only provides you with accurate temperature and humidity readings but also serves as a great foundation for more advanced environmental monitoring systems. As you become more comfortable with Arduino programming and sensor integration, you can continue to expand and customize your weather station to suit your specific needs – how about adding some automatic plant watering when it’s to hot?
Remember, the world of DIY electronics is all about experimentation and continuous learning. Happy building!