Are you someone who likes to ventilate your rooms but often forgets the windows open? This happens to me regularly – until now. In this project, an Arduino Nano monitors the temperature as soon as you open the window. If the temperature in the room drops by 0.5°C, it triggers an alarm. This way, you can ensure the room or even the entire apartment doesn’t cool down unnecessarily.
Components Needed:
- Arduino Nano
- DHT22 Temperature Sensor
- Piezo Buzzer
- Button
- 2x 10kΩ Resistors
- Breadboard & Cables
How the Temperature Monitoring Works
When you open the window, you press a button on the Arduino – this sets the initial temperature value, and measurement begins. As cooler air flows into the room (except in summer), the temperature will gradually drop. Once the room temperature drops by 0.5°C, the Piezo buzzer sounds an alarm to remind you to close the window. Press the button again: the alarm stops, and the Arduino resets to standby mode – until the next time you ventilate.
Of course, you can change the 0.5°C threshold to suit your preferences, the Arduino’s location in the room, etc.
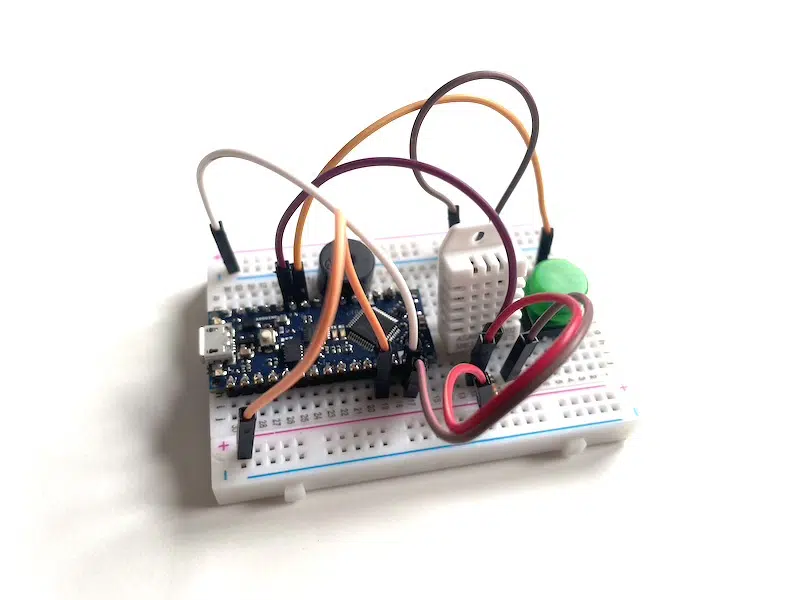
How to Assemble the Project
Follow the schematic below for assembly. I used an Arduino Nano for this project due to its compact size. However, you can use any other board you have on hand, such as an Arduino UNO.
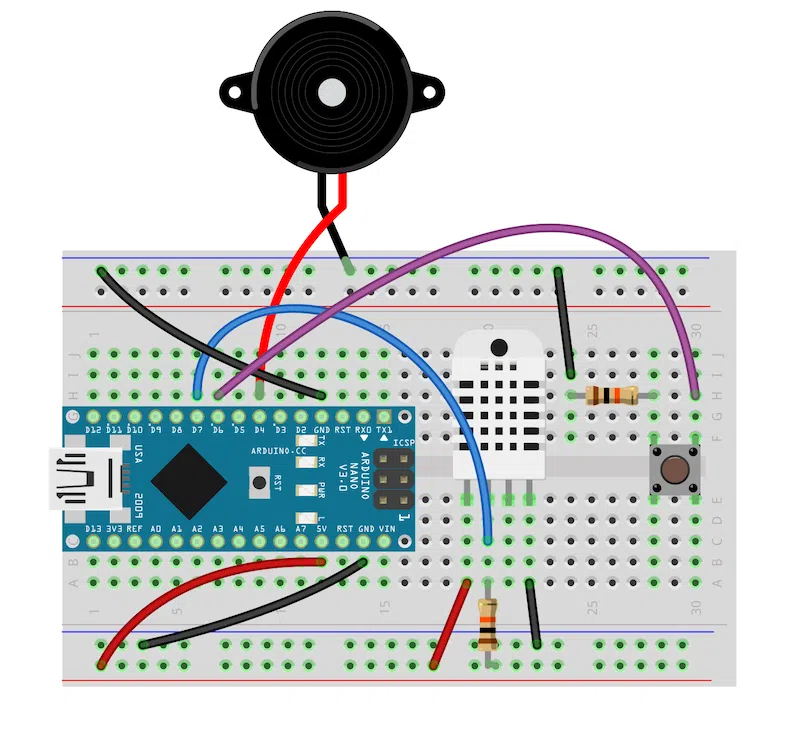
Once everything is wired, you can proceed with the sketch.
The Sketch for the Project
Before you copy and upload the following sketch to your Arduino, you’ll need the appropriate libraries for the DHT22 sensor – if you haven’t worked with this sensor in the Arduino IDE before.
In this case, open the Library Manager in the IDE, search for “DHT sensor library,” and click Install. You’ll be asked whether to also install the Adafruit Unified Sensor library in a dialog box. Confirm with Yes.
___STEADY_PAYWALL___
Now, here is the complete sketch:
#include <DHT.h>
// Pins
#define DHTPIN 7 // DHT22 on Pin D7
#define BUZZER_PIN 4 // Piezo Buzzer on Pin D4
#define BUTTON_PIN 6 // Button on Pin D6
#define LED_PIN 13 // Internal LED on Pin D13
// DHT Sensor
#define DHTTYPE DHT22
DHT dht(DHTPIN, DHTTYPE);
// Variables
float startTemperature = 0.0;
bool measuring = false;
bool alarmActive = false;
void setup() {
Serial.begin(9600);
dht.begin();
pinMode(BUZZER_PIN, OUTPUT);
pinMode(BUTTON_PIN, INPUT_PULLUP); // Enable internal pull-up resistor
pinMode(LED_PIN, OUTPUT);
digitalWrite(BUZZER_PIN, LOW);
digitalWrite(LED_PIN, LOW); // LED initially off
}
void loop() {
// Check button (toggle between measurement and idle mode)
if (digitalRead(BUTTON_PIN) == LOW) { // Button pressed
delay(50); // Debounce
if (digitalRead(BUTTON_PIN) == LOW) {
if (!measuring) {
// Start measurement
measuring = true;
alarmActive = false; // Ensure alarm is deactivated
startTemperature = dht.readTemperature();
if (isnan(startTemperature)) {
Serial.println("Error reading from DHT22!");
startTemperature = 0.0; // Default value
}
Serial.print("Measurement started. Start temperature: ");
Serial.println(startTemperature);
digitalWrite(LED_PIN, HIGH); // Turn on LED
} else {
// Switch to idle: stop alarm and measurement
noTone(BUZZER_PIN); // Stop alarm sound
digitalWrite(LED_PIN, LOW); // Turn off LED
alarmActive = false;
measuring = false;
Serial.println("Alarm deactivated and idle mode activated.");
}
while (digitalRead(BUTTON_PIN) == LOW);
delay(50); // Debounce after release
}
}
// Measurement logic, if active
if (measuring) {
float currentTemperature = dht.readTemperature();
if (isnan(currentTemperature)) {
Serial.println("Error reading from DHT22!");
delay(2000);
return;
}
// Check temperature change
if (currentTemperature <= startTemperature - 0.5) {
alarmActive = true; // Trigger alarm
}
// Execute alarm
if (alarmActive) {
tone(BUZZER_PIN, 1000); // Alarm tone
Serial.println("Alarm: Temperature has dropped!");
} else {
noTone(BUZZER_PIN); // Deactivate alarm tone
}
// Debug output
Serial.print("Current temperature: ");
Serial.println(currentTemperature);
delay(2000); // Measure every 2 seconds
}
}
How the Sketch Works
Let’s take a closer look at the sketch. First, you include the library for the temperature sensor and define the component pins:
#include <DHT.h>
#define DHTPIN 7 // DHT22 on Pin D7
#define BUZZER_PIN 4 // Piezo Buzzer on Pin D4
#define BUTTON_PIN 6 // Button on Pin D6
#define LED_PIN 13 // Internal LED on Pin D13
#define DHTTYPE DHT22
DHT dht(DHTPIN, DHTTYPE);
Next, the required variables are declared. The startTemperature
variable stores the temperature at the start of the measurement – i.e., when you press the button. The measuring
and alarmActive
variables control the program flow later.
float startTemperature = 0.0;
bool measuring = false;
bool alarmActive = false;
Setup Function
Here, you start the Serial Monitor and initialize the sensor. You also define the required pin modes and enable the Arduino’s internal pull-up resistor for the button. Lastly, ensure the Piezo buzzer and the internal LED are turned off:
void setup() {
Serial.begin(9600);
dht.begin();
pinMode(BUZZER_PIN, OUTPUT);
pinMode(BUTTON_PIN, INPUT_PULLUP); // Enable internal pull-up resistor
pinMode(LED_PIN, OUTPUT);
digitalWrite(BUZZER_PIN, LOW);
digitalWrite(LED_PIN, LOW); // LED initially off
}
Loop Function
This is the main part of the program, running continuously. Here, it determines whether to perform measurements or not:
if (digitalRead(BUTTON_PIN) == LOW) {
// If the button is pressed, start the measurement unless it's already active
}
When the button is pressed, the following occurs: if the measurement isn’t active (measuring
is false), it starts. The measuring
variable is then set to true.
If the measurement is already running, it stops. The alarm is deactivated, and the LED is turned off. The measuring
variable is reset to false, putting the Arduino in idle mode.
During measurement, it checks every 2 seconds whether the current temperature is more than 0.5°C below the start temperature. If so, it activates the alarm:
// Check temperature change
if (currentTemperature <= startTemperature - 0.5) {
alarmActive = true; // Trigger alarm
}
For this, the alarmActive
variable is set to true, which activates the Piezo buzzer in the following check:
if (alarmActive) {
tone(BUZZER_PIN, 1000); // Alarm tone
Serial.println("Alarm: Temperature has dropped!");
} else {
noTone(BUZZER_PIN); // Deactivate alarm tone
}
What’s Next?
You now have an Arduino that sounds an alarm whenever the temperature drops to your set threshold. Instead of a Piezo buzzer, you could also opt for another notification method. How about a push notification to your smartphone?